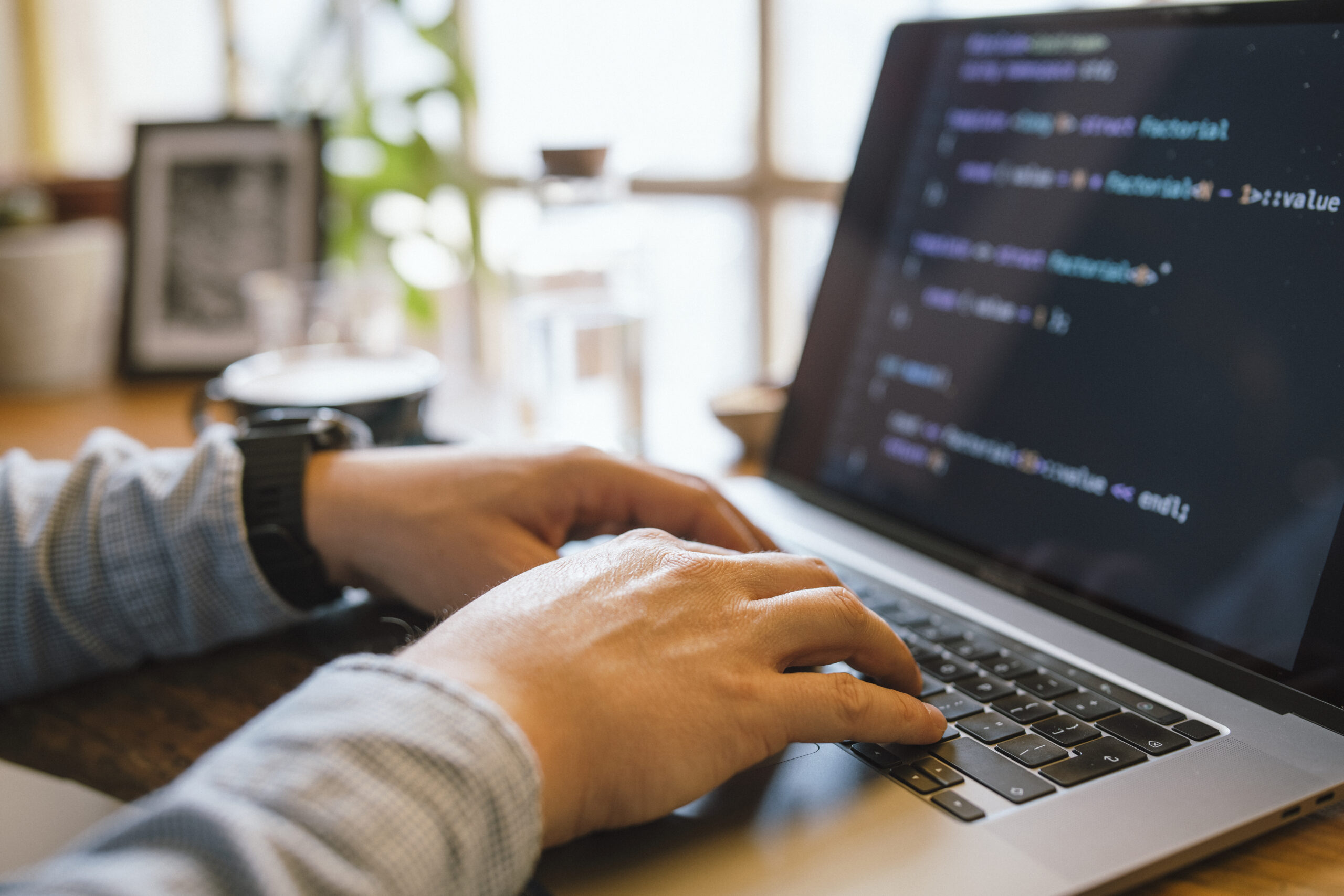
Debugging is Among the most important — nevertheless generally missed — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to resolve troubles successfully. Irrespective of whether you are a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and significantly enhance your productivity. Here are quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the resources they use every day. Though producing code is one particular Portion of advancement, realizing how to connect with it properly in the course of execution is equally significant. Modern day improvement environments occur Outfitted with effective debugging abilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Integrated Growth Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code on the fly. When applied properly, they Permit you to observe accurately how your code behaves in the course of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI troubles into manageable tasks.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Variation control methods like Git to know code historical past, come across the precise instant bugs were launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about building an intimate familiarity with your growth natural environment to make sure that when challenges crop up, you’re not lost in the dark. The greater you know your tools, the greater time you can spend resolving the particular challenge rather then fumbling as a result of the procedure.
Reproduce the situation
One of the more significant — and infrequently neglected — methods in powerful debugging is reproducing the trouble. Just before jumping into your code or building guesses, builders want to create a consistent ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Inquire queries like: What actions triggered the issue? Which ecosystem was it in — progress, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the less difficult it gets to be to isolate the exact conditions less than which the bug happens.
When you’ve gathered ample info, seek to recreate the trouble in your neighborhood setting. This could signify inputting precisely the same info, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, take into account creating automatic tests that replicate the edge conditions or condition transitions associated. These tests not simply assist expose the situation but additionally avert regressions in the future.
At times, The difficulty might be natural environment-particular — it would happen only on specific running units, browsers, or below distinct configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But when you can constantly recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more properly, take a look at possible fixes securely, and talk a lot more Obviously along with your staff or people. It turns an summary grievance into a concrete challenge — and that’s exactly where developers prosper.
Read and Comprehend the Error Messages
Error messages tend to be the most precious clues a developer has when anything goes Mistaken. As opposed to viewing them as irritating interruptions, builders should really study to deal with error messages as immediate communications with the process. They typically let you know precisely what transpired, wherever it took place, and often even why it occurred — if you know how to interpret them.
Begin by reading the information thoroughly and in complete. Many builders, particularly when below time tension, look at the initial line and immediately start out generating assumptions. But deeper during the mistake stack or logs might lie the true root bring about. Don’t just copy and paste mistake messages into serps — go through and understand them initially.
Break the mistake down into elements. Can it be a syntax error, a runtime exception, or a logic mistake? Does it position to a specific file and line range? What module or perform brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java typically abide by predictable designs, and Discovering to recognize these can considerably speed up your debugging course of action.
Some mistakes are imprecise or generic, and in People cases, it’s vital to look at the context by which the mistake occurred. Check out associated log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers serious-time insights into how an software behaves, encouraging you understand what’s happening beneath the hood while not having to pause execution or action from the code line by line.
A very good logging system starts off with knowing what to log and at what level. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic data for the duration of growth, Data for basic activities (like effective begin-ups), Alert for probable troubles that don’t split the appliance, ERROR for precise challenges, and Deadly if the technique can’t carry on.
Steer clear of flooding your logs with excessive or irrelevant facts. Far too much logging can obscure significant messages and slow down your system. Deal with critical activities, point out improvements, input/output values, and critical final decision factors inside your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace issues in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where stepping by code isn’t achievable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about balance and clarity. By using a well-considered-out logging method, you may reduce the time it will require to spot issues, achieve deeper visibility into your apps, and Increase the All round maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not only a complex task—it's a kind of investigation. To proficiently identify and correct bugs, builders should technique the procedure like a detective solving a mystery. This frame of mind allows break down advanced issues into manageable areas and observe clues logically to uncover the foundation cause.
Begin by collecting evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or overall performance issues. Much like a detective surveys a crime scene, gather as much related details as it is possible to with no leaping to conclusions. Use logs, examination situations, and consumer stories to piece jointly a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Check with on your own: What may very well be resulting in this habits? Have any changes not too long ago been created into the codebase? Has this difficulty happened right before below very similar instances? The intention would be to slender down alternatives and identify potential culprits.
Then, exam your theories systematically. Seek to recreate the situation in the controlled ecosystem. For those who suspect a selected operate or element, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, request your code queries and let the effects direct you closer to the reality.
Shell out near attention to compact aspects. Bugs generally cover within the the very least predicted sites—just like a lacking semicolon, an off-by-just one error, or a race affliction. Be comprehensive and patient, resisting the urge to patch The problem without thoroughly check here knowing it. Temporary fixes may well conceal the actual issue, just for it to resurface afterwards.
And finally, keep notes on Whatever you tried and uncovered. Just as detectives log their investigations, documenting your debugging approach can save time for long run difficulties and help Many others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach troubles methodically, and come to be more effective at uncovering hidden concerns in advanced systems.
Compose Assessments
Producing checks is one of the most effective strategies to help your debugging skills and General growth effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning changes for your codebase. A effectively-examined software is simpler to debug as it means that you can pinpoint exactly exactly where and when a difficulty happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glimpse, significantly reducing some time expended debugging. Unit exams are especially practical for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Next, combine integration exams and finish-to-end assessments into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re specifically useful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting assessments also forces you to Assume critically about your code. To check a characteristic properly, you may need to know its inputs, envisioned outputs, and edge circumstances. This volume of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails continually, you can target correcting the bug and view your take a look at go when the issue is resolved. This strategy makes sure that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a discouraging guessing activity into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your monitor for several hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks assists you reset your head, lessen irritation, and infrequently see the issue from the new standpoint.
If you're too close to the code for too lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your focus. Many builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Particularly during for a longer period debugging periods. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each individual Bug
Each and every bug you face is much more than simply A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking oneself a number of critical issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code reviews, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Establish much better coding behaviors transferring forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you figured out. After some time, you’ll begin to see patterns—recurring problems or popular issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast understanding-sharing session, helping Some others stay away from the same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. After all, many of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Improving your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.